“What’s the difference between an Event and a Delegate?”
Yes…The most popular question, which almost every one would have been asked during an interview.
In order to illustrate the difference we need to understand first that, What an Event is? and What a Delegate is?
What is an Event? Event is nothing but the action done on any control. e.g. Clicking a Button, Check-Un Check a Radio Button or a Check Box etc..
Suppose you have a simple form (ASP.NET/ WINDOWS) ame as below
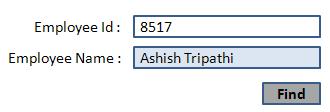
Its a simple search functionality form, on which you need enter Employee Id and you will be displayed corresponding Employee Name on click of Find button. When ever you Click on the “Find” button one method/piece of code (Where logic for displaying Emp Name is written) should be executed in order to display name of the employee.
Now the concept of delegates will come into picture. In fact in simple words delegate means an intermediate person who connects you to other. In the same way on your form when ever you do any action, then delegate only directs the event to that particular method.
In other words “Event is an Array List of delegates”.
What is a Delegate? You can understand delegates as a method which is capable of taking reference of any method/ function. Its similar to C++ function pointers.
Now some differences,
Events | Delegates |
Events are raised by using Deligates. | Deligate is a Function Pointer that stores address of any function. |
Events are the an action done on the control. | delegate is an intermediate which work between the control and the method. |
Event can fire more than one delegate, each delegate call one method. | Delegate can fire only one method. |
Event can fire more than one delegate, each delegate call one method. | Delegate can fire only one method. |
Implementation of Delegate
Again we would implement a very frequently asked interview question in order to understand the practical uses of delegate,
Question/ Scenario : You have a Window Form Application containing two Windows Forms say Parent and PopUp. Now you are suppose to open the PopUp Form from Parent Form. Once PopUp Form is opened, change the color of Parent Form by some action/ event on PopUp Form using Delegates.
Implementation:
- Create a Windows Application
- Add two Forms to the same (Parent and PopUp)
- Create a button on Parent Form say “Display Pop-Up”
- Write the code to bring the Pop-Up form
- Now, go to the PopUp Form, declare one delegate and an event which would be used to change the color of parent form
- Create a button on the PopUp Form say “Change Parent Color”
- Double click “Change Parent Color” button to write the event for the same
- Make a call to the to the event i.e. ColorChangerDelegate
private void btnSetParentColor_Click(object sender, EventArgs e)
{
ColorChangerDelegate(Color.Yellow);
}
- Now, we are done with PopUp Form by raising the event. Now, parent form is supposed to receive the event raised on PopUp Form and change its color.
- In order to change the color of parent we are supposed write the lines of code which we left in Step-4 with TODO comment
PopUp popUpWindow = new PopUp();
popUpWindow .Show();popUpWindow .ColorChangerDelegate +=new PopUp.ColorChanger(Change_Color);
- Now we are supposed to implement a method Change_Color which would change the color of parent
public void Change_Color(Color color)
{
this.BackColor = color;
}
i.e.
PopUp popUpWindow = new PopUp();
popUpWindow.Show();
/*
TODO : HERE WE WILL WRITE CODE TO CHANGE THE COLOR OF PARENT
*/
public delegate void ColorChanger(Color color);
public event ColorChanger ColorChangerDelegate;
Note: Please do not hesitate if you require the source code or more information. I would be reachable to ak.tripathi@yahoo.com